Data Structures and Their Functions in Python
Introduction: Data structures are fundamental components of programming languages like Python. They serve as the building blocks for organizing, storing, and manipulating data efficiently. In Python, a versatile and widely-used programming language, various data structures are available, each with its own unique characteristics and functions. This article explores some of the most common data structures in Python and delves into their functions, use cases, and advantages.
1. Lists:
Lists in Python are one of the most versatile and frequently used data structures. They are ordered collections of elements and are defined using square brackets. Lists can contain elements of different data types, making them highly flexible.
Functions:
- Appending and Extending: Lists can be modified by adding elements to the end. The append() method adds a single element, while the extend() method adds multiple elements.
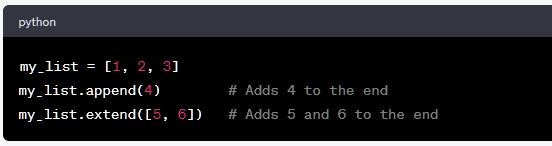
Indexing and Slicing: You can access elements of a list using their index, and you can also slice a list to retrieve a portion of it.
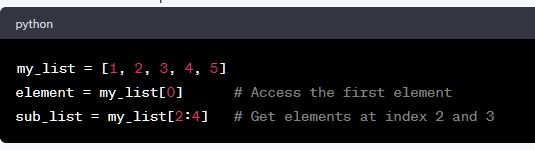
Searching and Counting: Python lists provide methods like index()
and count()
to find the index of a specific element and count occurrences of an element, respectively.
Sorting and Reversing: Lists can be sorted using the sort()
method, and their order can be reversed using reverse()
.
2. Tuples:
Tuples are similar to lists but with a crucial difference: they are immutable. This means once you create a tuple, you cannot change its elements. Tuples are defined using parentheses.
Functions:
- Packing and Unpacking: You can create tuples by packing multiple values together and unpack them into individual variables.
- Accessing Elements: You can access tuple elements using indexing, just like in lists.
- Immutability: Since tuples are immutable, they are suitable for storing data that should not change during program execution.
- Tuple Concatenation and Repetition: You can concatenate tuples using the
+
operator and repeat them using the*
operator. - Set Operations: Sets support various set operations like union, intersection, and difference.
- Checking Membership: You can check if an element is present in a set using the
in
keyword. - Set Comprehensions: Like list comprehensions, Python also supports set comprehensions for creating sets in a concise way.
4. Dictionaries:
Dictionaries in Python are collections of key-value pairs. They are unordered, and keys must be unique. Dictionaries are defined using curly braces {}
or the dict()
constructor.
Functions:
- Adding and Updating Elements: You can add or update elements in a dictionary by assigning values to keys.
- Accessing Elements: You can access dictionary values using their keys.
- Removing Elements: You can remove elements from a dictionary using the
pop()
method or thedel
statement. - Iterating Over Dictionaries: You can iterate over keys, values, or key-value pairs in a dictionary using loops.